The Document Object Model JS Home << JS Advanced << Document Object Model
When we view a web page, the page has been loaded up by our web browser which displays the contents of our web file by interrogating the HTML we have supplied and applying any CSS. As the web browser builds our page it builds up a model of the page's HTML tags and any attributes and values attached to each HTML tag. It also remembers the order in which they appear in the HTML file and treats each individual entry (html, attribute or text) as a node. This model hierarchy is known as the Document Object Model, more commonly known as the DOM?
So what has this to do with JavaScript? Well the DOM supplies us with the information required to communicate with the HTML elements of our web pages. Before explaining how to do this let's look at the basic structure of some HTML as a family tree, with the <html> element at the root.
Basic HTML Structure
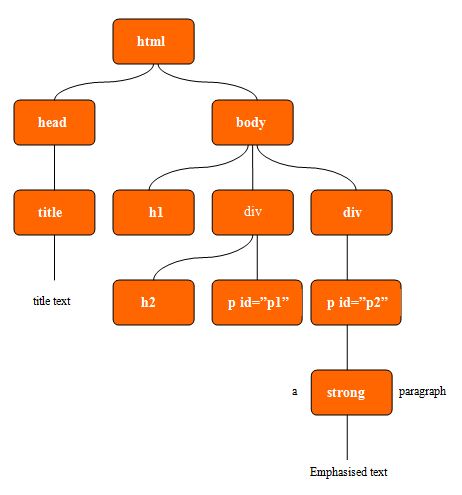
Accessing the DOM
Let's see how we can use the DOM to access the disparate parts of a HTML tree. We access the DOM nodes by using various methods contained with the document object supplied by our web browsers. These methods allow us to use JavaScript on the node acquired to add dynamics to our pages.
Accessing Individual Elements Using getElementById()
Method
The getElementById()
method allows us to acquire a DOM node using the value of a CSS id selector. So using the diagram above as an example we could retrieve the two paragraphs marked with the id selector using
a literal string or a variable. We can also manipulate the contents of an element we pick, following are examples:
// retrieve using literal string
var idNodeA = document.getElementById('p1');
// retrieve using a variable
var idNodeB = 'p2');
var findNode = document.getElementById(idNodeB;)
// Access h4 heading above and change the colour
var changeh4TextColour = document.getElementById('h4tag');
changeh4TextColour.style.color = 'red';
Accessing Element Types Using getElementsByTagName()
Method
When we want to access all the elements of a certain type we can use the getElementsByTagName()
method. This method traverses the DOM
looking for the specified HTML element and returns a list of nodes found. The returned list is an array-like object and can be accessed as such starting from the zero index. So using the diagram above as an example we
could retrieve all the <div> elements as follows:
var getDivs = document.getElementsByTagName('div');
var totalDivs = getDivs.length;
/*
* Access all <p> tags on page and change the colour
* of a range of paragraphs to green
*/
var changepTextColour = document.getElementsByTagName('p');
for (i=5;i<9;i++) {
changepTextColour[i].style.color = 'green';
}
We can use the getElementById()
and getElementsByTagName()
methods in tandem to refine our search. Following is an example of this:
// retrieve element by id
var idNodeA = document.getElementById('p2');
// retrieve <strong> elements with this paragraph
var getStrong = idNodeA.getElementsByTagName('strong');
Selecting Relative Nodes
The DOM also allows us to select relative nodes in relation to a node we have selected previously. We can select parent, child and sibling nodes as illustrated below.
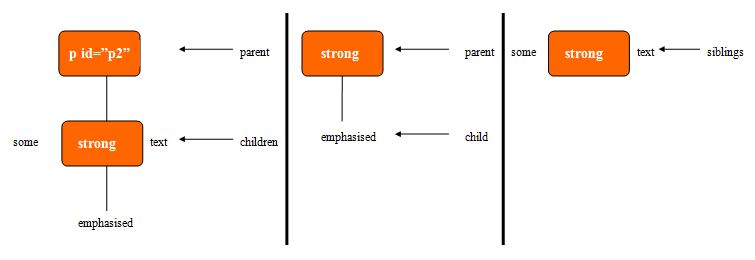
Let's look at some examples of using relative node selection.
// retrieve element by id
var idNodeA = document.getElementById('p2');
// retrieve child nodes
var childNodes = idNodeA.childNodes;
// retrieve parent nodes
var childNodes = idNodeA.parentNode;
// retrieve previous and next sibling
var prevSibling = idNodeA.prevSibling;
var nextSibling = idNodeA.nextSibling;
Modifying the DOM with the innerHTML
property
Traversing the DOM is a time consuming and laborious task which is exascerbated when we want to add to or modify the DOM. Fortunately web browsers provide the innerHTML
property which represents all the
HTML contained within a particular node. To illustrate how to use the innerHTML
property the following code will change the above heading title
// get all h3 elements
var modifyh3 = document.getElementById('modh3');
modifyh3.innerHTML = 'Modifying DOM with the innerHTML property MODIFIED'
Lesson 6 Complete
In this lesson we looked at the DOM and how to access and manipulate elements using the DOM and JavaScript.